Project Overview:
This project uses an Arduino to monitor gas levels with a gas sensor. Based on the detected gas concentration, different LEDs light up to indicate safe, warning, or dangerous levels of gas in the environment.
Components List:
- Arduino Uno (or compatible)
- 1 x Gas Sensor (e.g., MQ-2)
- 1 x Green LED
- 1 x Yellow LED
- 2 x Red LEDs
- 4 x 220Ω Resistors (for the LEDs)
- Breadboard and Jumper Wires
Circuit Connection:
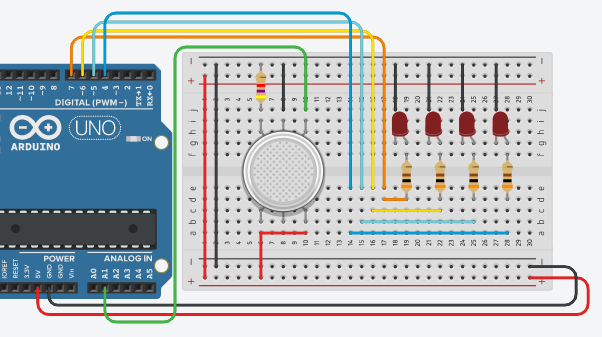
- Gas Sensor:
- Connect the analog output pin of the gas sensor to the Arduino’s analog pin A1.
- LEDs Setup:
- Connect the Green LED to digital pin 7.
- Connect the Yellow LED to digital pin 6.
- Connect the first Red LED to digital pin 5.
- Connect the second Red LED to digital pin 4.
- Place a 220Ω resistor in series with each LED to limit the current.
Project Code:
int PINO_SGAS = A1; // Pin connected to gas sensor
int LED_VERDE = 7; // Green LED pin
int LED_AMARELO = 6; // Yellow LED pin
int LED_VERMELHO1 = 5; // First Red LED pin
int LED_VERMELHO2 = 4; // Second Red LED pin
void setup() {
pinMode(LED_VERDE, OUTPUT); // Set LED pins as outputs
pinMode(LED_AMARELO, OUTPUT);
pinMode(LED_VERMELHO1, OUTPUT);
pinMode(LED_VERMELHO2, OUTPUT);
}
void loop() {
int valor = analogRead(PINO_SGAS); // Read the gas sensor value
valor = map(valor, 300, 750, 0, 100); // Map sensor value to a percentage (0-100)
// Control the LEDs based on the mapped value
digitalWrite(LED_VERDE, HIGH); // Green LED always on
digitalWrite(LED_AMARELO, valor >= 30 ? HIGH : LOW); // Yellow LED on if value >= 30
digitalWrite(LED_VERMELHO1, valor >= 50 ? HIGH : LOW); // First Red LED on if value >= 50
digitalWrite(LED_VERMELHO2, valor >= 80 ? HIGH : LOW); // Second Red LED on if value >= 80
delay(250); // Small delay to prevent flickering
}
Explanation of the Code:
- Setup Function:
- Configures the LED pins as outputs.
- Loop Function:
- Reads the analog value from the gas sensor and maps it to a range between 0 and 100.
- The Green LED is always on, indicating the system is running.
- The Yellow LED lights up if the gas level reaches 30% or higher, indicating a warning.
- The first Red LED lights up if the gas level reaches 50% or higher, signaling a potential danger.
- The second Red LED lights up if the gas level reaches 80% or higher, indicating a critical situation.
Summary:
This project is useful in environments where gas leakage detection is critical. The LEDs provide a visual indication of the gas levels, allowing for quick response in case of dangerous concentrations. The setup can be modified for different gas sensors and thresholds depending on the application.
No comments