Materials Required:
- Arduino Nano (Any version will work)
- Ultrasonic Sensor HC-SR04
- LDR
- Buzzer and LED
- 7805
- 433MHz RF transmitter and receiver
- Resistors
- Push button
- Perf board
- Soldering Kit
- 9V batteries
Circuit Diagram:
This Arduino Smart Blind Stick Project requires two separate circuits. One is the main circuit which will be mounted on the blind man’s stick. The other is a small remote RF transmitter circuit which will be used to locate the main circuit. The main board’s circuit diagram is shown below:
As we can see an Arduino Nano is used to control all the sensors. The complete board is powered by a 9V battery which is regulated to +5V using a 7805 Voltage regulator. The Ultrasonic sensor is powered by 5V and the trigger and Echo pin is connected to Arduino nano pin 3 and 2 as shown above. The LDR is connected with a resistor of value 10K to form a Potential divider and the difference in voltage is read by Arduino ADC pin A1. The ADC pin A0 is used to read the signal from RF receiver. The output of the board is given by the Buzzer which is connected to pin 12.
The RF remote circuit is shown below. Its working is also further explained.
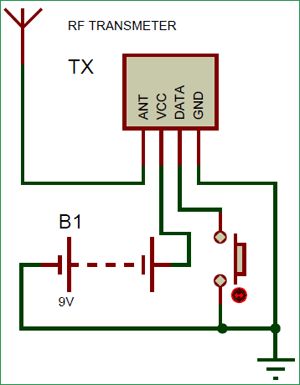
I have used a small hack to make this RF remote control circuit to work. Normally while using this 433 MHz module requires an Encoder and Decoder or two MCU to work. But, in our application we just need the receiver to detect if the transmitter is sending some signals. So the Data pin of the transmitter is connected to Ground or Vcc of the supply.
The data pin of the receiver is passed through an RC filter and then given to the Arduino as shown below. Now, whenever the button is pressed the Receiver output some constant ADC value repeatedly. This repetition cannot be observed when the button is not pressed. So we write the Arduino program to check for repeated values to detect if the button is pressed.
I have used a perf board to solder all the connections so that it gets intact with the stick. But, you can also make them on a breadboard. The boards that I made are below.
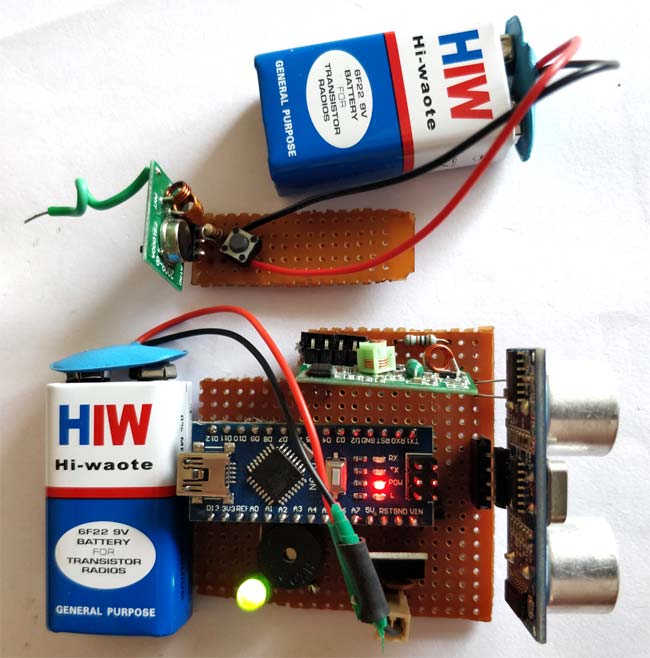
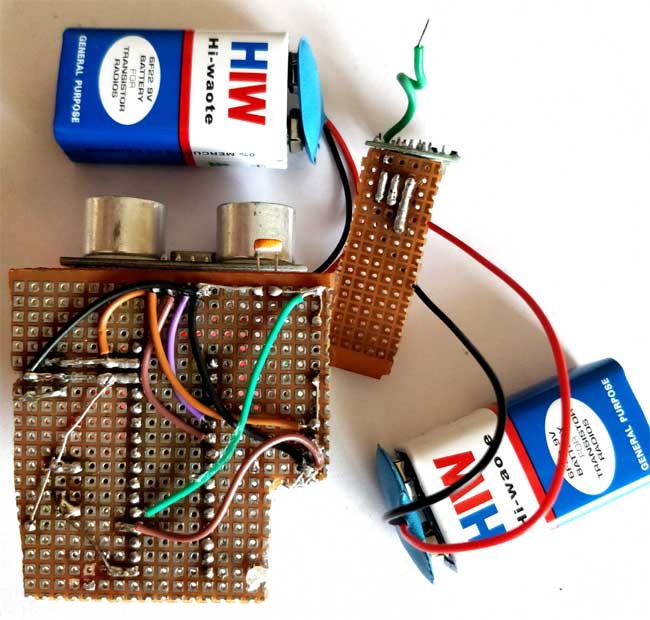
Arduino Program for Smart Blind Stick:
Once we are ready with our hardware, we can connect the Arduino to our computer and start programming. The complete code used for this page can be found at the bottom of this page. However, if you are curious to know how the code works read further.Like all programs we start with void setup () to initialize Input Output pins. In our program the Buzzer and Trigger is an Output device and the Echo pin is an Input device. We also start the serial monitor for debugging.
void setup ()
{
Serial.begin (9600);
pinMode (Buzz, OUTPUT);
digitalWrite (Buzz, LOW);
pinMode (trigger, OUTPUT);
pinMode (echo, INPUT);
}
Inside the main loop we are reading all the sensors data. We may begin with the sensor data of Ultrasonic sensor for distance, LDR for light intensity and RF signal to check if the button is pressed. All these data is saved as a future.
calculate_distance (trigger, echo);
Signal = analogRead (Remote);
Intens = analogRead (Light);
We will start with the Remote signal. We use a variable called similar_count to check how many times the same values are being repeated from the RF receiver. This repetition will occur only when the button is pressed. So we trigger the Remote pressed alarm if the count exceeds a value of 100
// Check if Remote is pressed
int temp = analogRead (remote);
similar_count = 0;
while (Signal == temp)
{
Signal = analogRead (Remote);
similar_count ++;
}
// If remote pressed
if (similar_count <100)
{
Serial.print (similar_count); Serial.println ("Remote Pressed");
digitalWrite (Buzz, HIGH); delay (3000); digitalWrite (Buzz, LOW);
}
You can also check it on Serial Monitor on your computer:
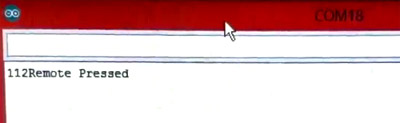
Next to the blind man If the LDR offers a value of less than 200 it is assumed to be very dark and we give him the warning through a special tone of delay with 200ms. If the intensity is very bright that is more than 800 then we give a warning with another tone The alarm tone and intensity can be easily varied by changing the relative value in the below code.
// If very dark
if (Intens <200)
{
Serial.print (Intens); Serial.println ("Bright Light");
digitalWrite (Buzz, HIGH); delay (200); digitalWrite (Buzz, LOW); delay (200); digitalWrite (Buzz, HIGH); delay (200); digitalWrite (Buzz, LOW); delay (200);
delay (500);
}
// If very bright
if (Intens> 800)
{
Serial.print (Intens); Serial.println ("Low Light");
digitalWrite (Buzz, HIGH); delay (500); digitalWrite (Buzz, LOW); delay (500); digitalWrite (Buzz, HIGH); delay (500); digitalWrite (Buzz, LOW); delay (500);
}
}
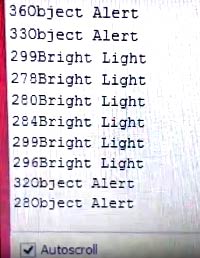
Finally, we start measuring the distance from any obstacle. There will be no alarm if the measured distance is more than 50cm. But, if it is less than 50cm the alarm will start by beeping the buzzer. As the object gets closer to the buzzer the beeping interval will also decrease. The closer the object is the faster the buzzer will beep. This can be done by creating a delay that is proportional to the distance measured. Since the delay () in Arduino cannot accept variables we have to use a for loop which loop based on the measured distance as shown below.
if (dist <50)
{
Serial.print (dist); Serial.println ("Object Alert");
digitalWrite (Buzz, HIGH);
for (int i = dist; i> 0; i--)
delay (10);
digitalWrite (Buzz, LOW);
for (int i = dist; i> 0; i--)
delay (10);
}
full code
const int trigger = 3; // Trigger pin of 1st Sesnor
const int echo = 2; // Echo pin of 1st Sesnor
const int buzz = 13; // Echo pin of 1st Sesnor
const int remote = A0; // Echo pin of 1st Sesnor
const int Light = A1; // Echo pin of 1st Sesnor
long time_taken;
int dist;
int Signal;
int Intens;
int similar_count;
void setup () {
Serial.begin (9600);
pinMode (Buzz, OUTPUT);
digitalWrite (Buzz, LOW);
pinMode (trigger, OUTPUT);
pinMode (echo, INPUT);
}
/ * ### Function to calculate distance ### * /
void calculate_distance (int trigger, int echo)
{
digitalWrite (trigger, LOW);
delayMicroseconds (2);
digitalWrite (trigger, HIGH);
delayMicroseconds (10);
digitalWrite (trigger, LOW);
time_taken = pulseIn (echo, HIGH);
dist = time_taken * 0.034 / 2;
if (dist> 300)
dist = 300;
}
void loop () {// infinite loopy
calculate_distance (trigger, echo);
Signal = analogRead (Remote);
Intens = analogRead (Light);
// Check if Remote is pressed
int temp = analogRead (remote);
similar_count = 0;
while (Signal == temp)
{
Signal = analogRead (Remote);
similar_count ++;
}
// If remote pressed
if (similar_count <100)
{
Serial.print (similar_count); Serial.println ("Remote Pressed");
digitalWrite (Buzz, HIGH); delay (3000); digitalWrite (Buzz, LOW);
}
// If very dark
if (Intens <200)
{
Serial.print (Intens); Serial.println ("Bright Light");
digitalWrite (Buzz, HIGH); delay (200); digitalWrite (Buzz, LOW); delay (200); digitalWrite (Buzz, HIGH); delay (200);
digitalWrite (Buzz, LOW); delay (200);
delay (500);
}
// If very bright
if (Intens> 800)
{
Serial.print (Intens); Serial.println ("Low Light");
digitalWrite (Buzz, HIGH); delay (500); digitalWrite (Buzz, LOW); delay (500); digitalWrite (Buzz, HIGH); delay (500);
digitalWrite (Buzz, LOW); delay (500);
}
if (dist <50)
{
Serial.print (dist); Serial.println ("Object Alert");
digitalWrite (Buzz, HIGH);
for (int i = dist; i> 0; i--)
delay (10);
digitalWrite (Buzz, LOW);
for (int i = dist; i> 0; i--)
delay (10);
}
//Serial.print("dist= ");
//Serial.println(dist);
//Serial.print("Similar_count= ");
//Serial.println(similar_count);
//Serial.print("Intens= ");
//Serial.println(Intens);
}
No comments